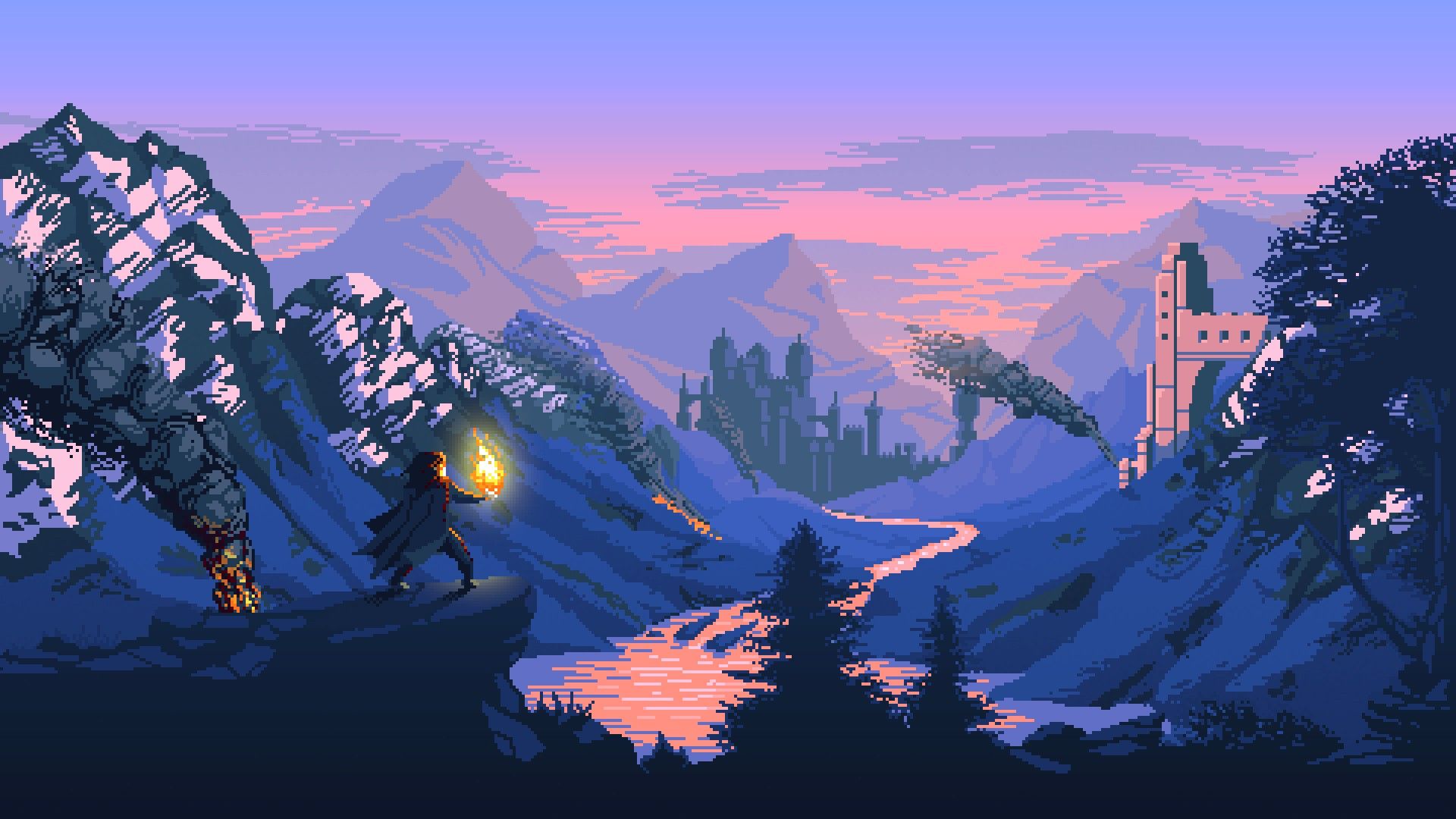
Welcome, cyber guardians and machine learning enthusiasts! Are you ready to delve deeper into the realm of artificial intelligence to combat malware? This guide is designed to take you through a comprehensive journey of detecting malware using machine learning, offering detailed insights into each step of the process.
In-Depth Analysis of Malware Detection
Malware detection using machine learning isn’t just about running algorithms; it’s a meticulous process that involves understanding the data, selecting the right models, and fine-tuning them for optimal performance.
The Significance of Data in Malware Detection
Data is the cornerstone of any machine learning project. In the context of malware detection, it’s imperative to have a dataset that accurately represents the types of malware you’re aiming to detect, along with benign software for comparison.
Deep Dive into the Dataset
After mounting our Google Drive to access the dataset, we embark on a thorough exploration of the data:
from google.colab import drive
drive.mount('/content/drive')
import pandas as pd
data = pd.read_csv('/content/drive/My Drive/uci_malware_detection.csv')
data.head()
This initial peek into the dataset allows us to understand its structure and the features involved. Each feature in this dataset represents some characteristic of the files, which could be binary content, metadata, behavioral data, or other attributes that help distinguish between benign and malicious files.
Cleaning and Preprocessing the Data
Ensuring our data is clean and appropriately formatted is crucial:
data.isnull().sum()
This command helps us identify if there are any missing values in our dataset, which is critical for maintaining the integrity of our machine learning model. Following this, we plot the distribution of malware and benign samples:
import seaborn as sns
sns.countplot(x='Label', data=data)
Visualizing the data distribution aids in understanding the balance between malware and benign samples, which is vital for training a model that can generalize well.
Model Selection and Training
Selecting the right model is pivotal in machine learning. Our approach involves experimenting with various algorithms to determine the most effective one for malware detection.
Exploring Different Machine Learning Models
We engage with a suite of machine learning models to find the best fit for our data:
from sklearn.model_selection import train_test_split
from sklearn.metrics import accuracy_score, confusion_matrix, classification_report
from sklearn.tree import DecisionTreeClassifier
from sklearn.ensemble import RandomForestClassifier, AdaBoostClassifier, ExtraTreesClassifier
from sklearn.neighbors import KNeighborsClassifier
from sklearn.linear_model import SGDClassifier
from sklearn.naive_bayes import GaussianNB
X = data.drop('Label', axis=1)
y = data['Label']
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
Here, train_test_split
is used to divide the data into training and testing sets, ensuring that our model is tested on unseen data, which is a good practice for evaluating model performance.
Training and Evaluating Models
For each model, we perform training and evaluation, assessing its accuracy and ability to generalize:
models = [DecisionTreeClassifier, RandomForestClassifier, KNeighborsClassifier, AdaBoostClassifier, SGDClassifier, ExtraTreesClassifier, GaussianNB]
accuracy_test = []
model_names = []
for model in models:
clf = model()
clf.fit(X_train, y_train)
predictions = clf.predict(X_test)
accuracy = accuracy_score(y_test, predictions)
accuracy_test.append(accuracy)
model_names.append(model.__name__)
print(f'Model: {model.__name__}, Accuracy: {accuracy:.2f}')
print(classification_report(y_test, predictions))
sns.heatmap(confusion_matrix(y_test, predictions), annot=True, fmt='.2f')
plt.show()
In this loop, each model is instantiated, trained on the training set, and then used to make predictions on the test set. The accuracy, classification report, and confusion matrix are displayed for each model, providing a comprehensive view of each model’s performance.
Comparative Analysis and Model Selection
After training, we compare the models to select the one that performs the best in terms of accuracy and generalization to unseen data.
Visualizing Model Performance
A comparative visualization of each model’s accuracy helps in making an informed decision about which model to choose for further tuning and deployment:
import matplotlib.pyplot as plt
output = pd.DataFrame({'Model': model_names, 'Accuracy': accuracy_test})
sns.barplot(x='Model', y='Accuracy', data=output)
plt.xticks(rotation=45)
plt.show()
This bar chart provides a clear and concise comparison of each model’s accuracy, guiding us in selecting the most effective model for detecting malware in our dataset.
Learn How To Build AI Projects
Learn How To Build AI Projects
Now, if you are interested in upskilling in 2024 with AI development, check out this 6 AI advanced projects with Golang where you will learn about building with AI and getting the best knowledge there is currently. Here’s the link.
Conclusion
Diving deep into the process of detecting malware using machine learning has uncovered the intricacies and challenges involved. By meticulously analyzing the data, experimenting with various models, and evaluating their performance, we have gained valuable insights into the art and science of machine learning in cybersecurity. This journey doesn’t end here; continue to explore, experiment, and evolve your models to stay ahead in the ever-changing landscape of cyber threats.