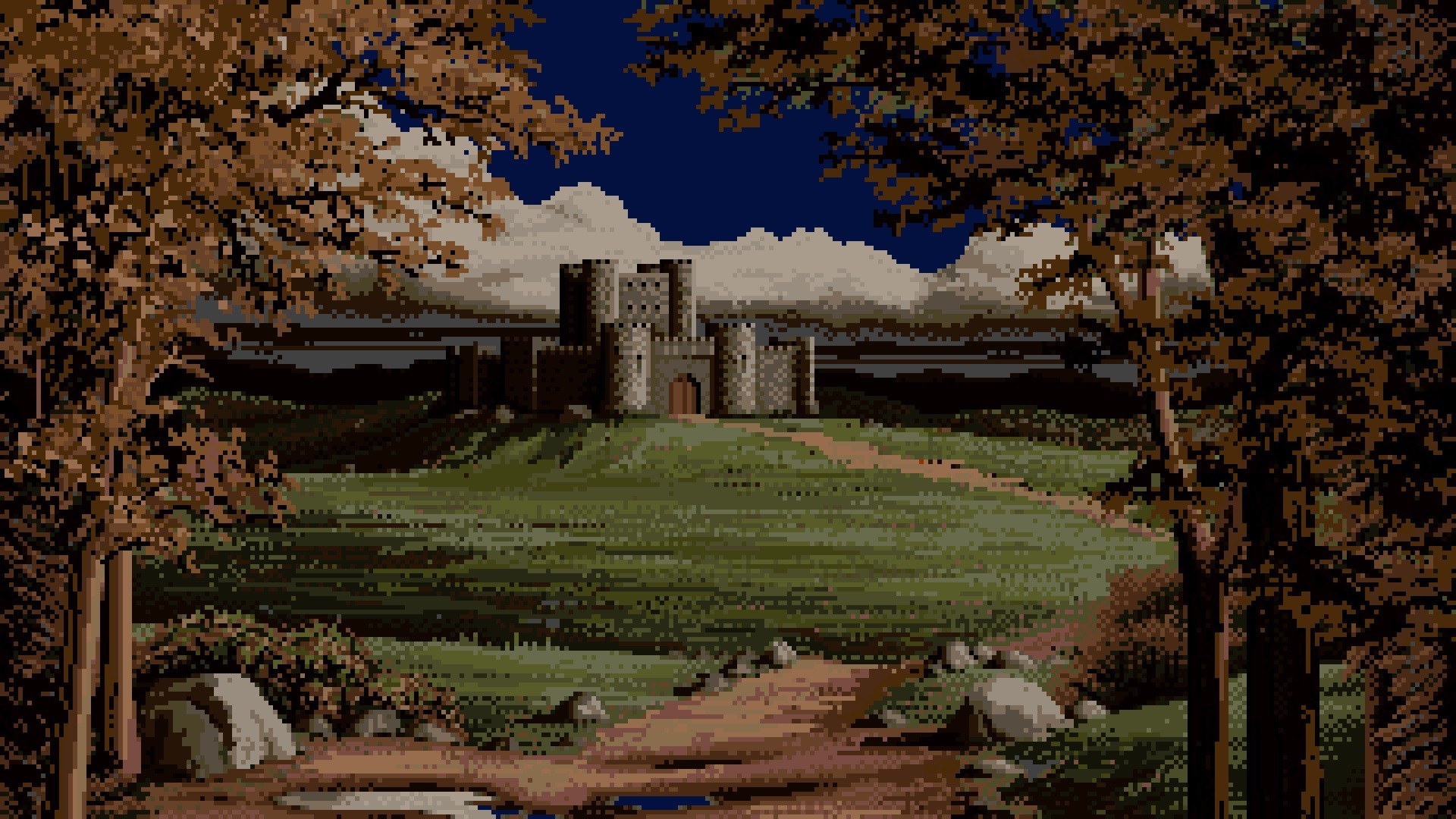
Welcome to our detailed guide on setting up and using the Idefics-9b bot! This project involves a potent vision-text transformer model capable of generating text from images combined with textual prompts. Whether you’re a developer, a student, or an AI enthusiast, this tutorial will provide you with a thorough understanding of each component and how they work together to achieve impressive results.
In this post, we’ll walk through the entire process of setting up the environment, understanding the code, and deploying a web-based interface to interact with the model. Our goal is to empower you with the knowledge to replicate this project and harness the power of AI in your applications.
What You Will Need
To follow along with this tutorial, you’ll need:
- A Python environment
- Access to a GPU (recommended for faster processing)
- Basic understanding of Python and neural networks
Step-by-Step Installation
Install Necessary Libraries:
Start by installing the required Python libraries. The Idefics-9b bot relies on several advanced libraries to handle large model operations and interface creation:
!pip install -q git+https://github.com/huggingface/transformers !pip install -q bitsandbytes sentencepiece accelerate loralib gradio !pip install -q -U git+https://github.com/huggingface/peft.git
These commands install libraries for handling transformer models (
transformers
), managing efficient floating point operations (bitsandbytes
), preprocessing text (sentencepiece
), speeding up operations (accelerate
), and creating a user interface (gradio
).
Understanding the Code
Import Libraries and Set Up the Environment:
import torch from PIL import Image from transformers import IdeficsForVisionText2Text, AutoProcessor import gradio as gr import requests from io import BytesIO device = "cuda" if torch.cuda.is_available() else "cpu"
This section loads necessary libraries and sets the computing device. If a GPU is available, it uses
cuda
for faster computation.Load the Model:
checkpoint = "HuggingFaceM4/idefics-9b" model = IdeficsForVisionText2Text.from_pretrained(checkpoint, torch_dtype=torch.bfloat16).to(device) processor = AutoProcessor.from_pretrained(checkpoint)
Here, we load the Idefics-9b model and its associated processor. The model is set to use bfloat16 precision to optimize GPU memory usage.
Define the Input and Generation Process:
prompts = [ [ "https://upload.wikimedia.org/wikipedia/commons/8/86/Id%C3%A9fix.JPG", "In this picture from Asterix and Obelix, we can see" ], ] inputs = processor(prompts, return_tensors="pt").to(device) bad_words_ids = processor.tokenizer(["<image>", "<fake_token_around_image>"], add_special_tokens=False).input_ids generated_ids = model.generate(**inputs, bad_words_ids=bad_words_ids, max_length=100) generated_text = processor.batch_decode(generated_ids, skip_special_tokens=True) for i, t in enumerate(generated_text): print(f"{i}:\n{t}\n")
This part of the code processes an image and accompanying text to generate descriptive text based on the input. It avoids generating specific unwanted tokens by setting
bad_words_ids
.Set Up the Gradio Interface:
def generate_text_from_image(image_url, text_prompt): response = requests.get(image_url) image = Image.open(BytesIO(response.content)) prompts = [[image, text_prompt]] inputs = processor(prompts, return_tensors="pt").to(device) generated_ids = model.generate(**inputs, bad_words_ids=bad_words_ids, max_length=100) generated_text = processor.batch_decode(generated_ids, skip_special_tokens=True) return generated_text[0], image_url
iface = gr.Interface(
fn=generate_text_from_image,
inputs=[gr.Textbox(label="Image URL", placeholder="Enter Image URL Here..."), gr.Textbox(label="Text Prompt", placeholder="Enter Text Prompt Here...")],
outputs=[gr.Textbox(label="Generated Text"), gr.Image(label="Input Image")],
title="Generate Text from Image and Text Prompt",
description="Enter an image URL and a text prompt to generate descriptive text. The image will be displayed alongside the generated text."
)
iface.launch()
The final step involves setting up a Gradio interface, which allows users to input an image URL and a text prompt, and see the model’s output in real-time. This makes the model interactive and user-friendly.
Conclusion
By following this guide, you now have a fully functional Idefics-9b bot that can generate descriptive texts from images and text prompts. This project not only showcases the power of combining vision and text data but also demonstrates how you can build advanced AI models into your applications.
Experiment with different images and prompts to see how the model performs across various scenarios. The possibilities are endless, and now you have the tools to explore them! Keep pushing the boundaries of what’s possible with AI.
Learn How To Build AI Projects
Learn How To Build AI Projects
Now, if you are interested in upskilling in 2024 with AI development, check out this 6 AI advanced projects with Golang where you will learn about building with AI and getting the best knowledge there is currently. Here’s the link.