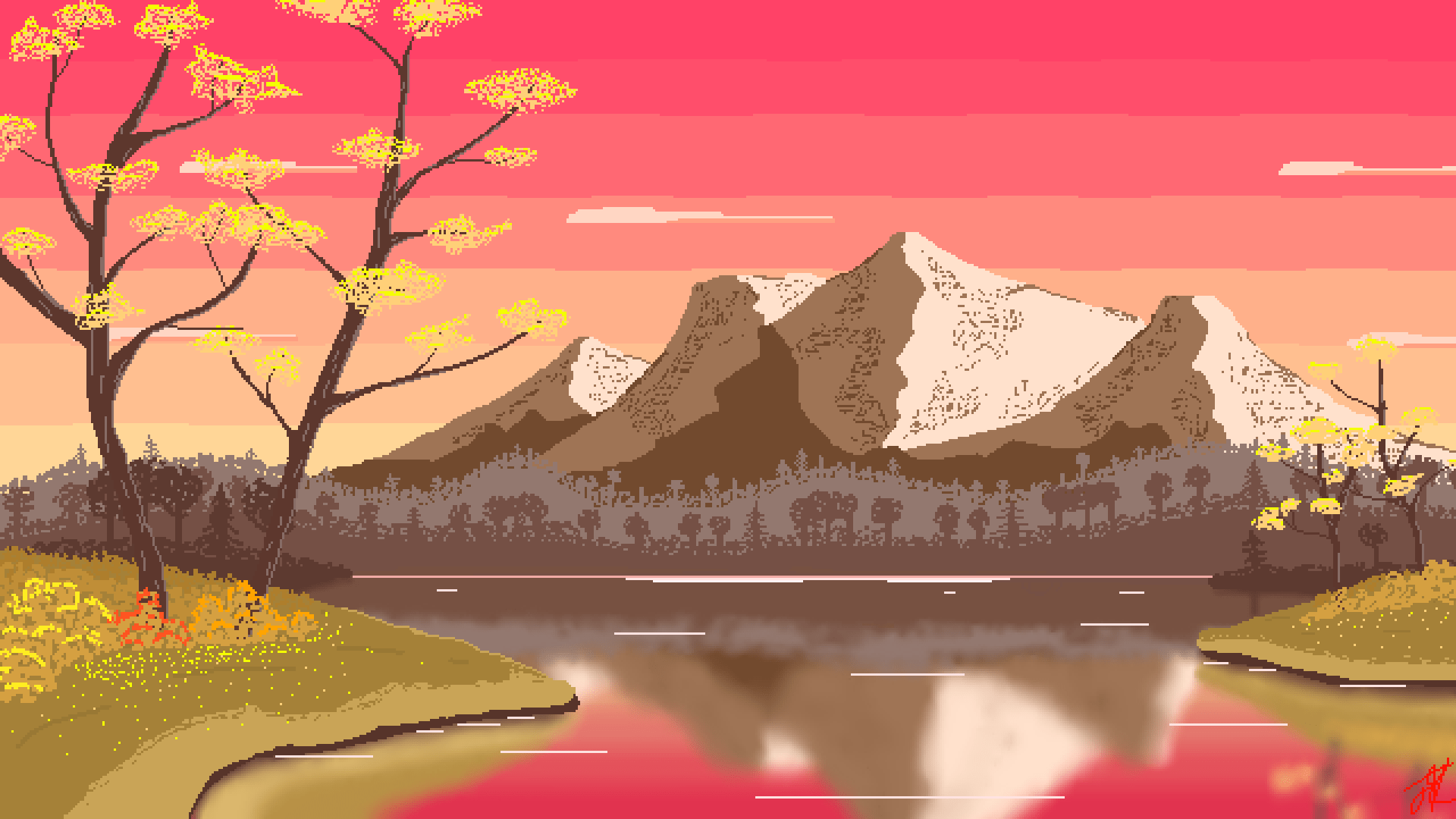
In a world where security and personalization are paramount, face recognition technology has emerged as a game-changer. Python, with its simplicity and robust libraries, offers a gateway into this fascinating world. Today, we’re going to explore how you can develop your own face recognition system using Python. By the end of this guide, you’ll not only understand the nuts and bolts of the process but also have a working project to show for it.
Getting Started: The Setup
To kick things off, ensure you have Python installed on your machine. We’ll be using libraries like OpenCV, imutils, and numpy, so make sure these are installed too (pip install opencv-python imutils numpy
). For this project, we’re also utilizing Google Colab for its convenience and powerful features, perfect for handling image processing tasks.
Understanding the Code
The journey begins with capturing an image. Here’s how we do it in a Google Colab environment:
import imutils
import numpy as np
import cv2
from google.colab.patches import cv2_imshow
def take_photo(filename='photo.jpg', quality=0.8):
# JavaScript to trigger the camera
# Code omitted for brevity
display(js)
data = eval_js('takePhoto({})'.format(quality))
binary = b64decode(data.split(',')[1])
with open(filename, 'wb') as f:
f.write(binary)
return filename
image_file = take_photo()
This function triggers the webcam, captures a photo, and saves it. The magic happens in the JavaScript part (omitted for brevity), which manages the camera interaction in the browser.
Next, we load and resize the image to prepare it for face detection:
image = cv2.imread(image_file)
image = imutils.resize(image, width=400)
cv2_imshow(image)
Here, imutils.resize
ensures the image is a manageable size, maintaining the aspect ratio.
Face Detection Mechanics
For face detection, we leverage a pre-trained deep learning model:
!wget -N [model and prototxt URLs]
prototxt = 'deploy.prototxt'
model = 'res10_300x300_ssd_iter_140000.caffemodel'
net = cv2.dnn.readNetFromCaffe(prototxt, model)
We download the model and configuration file, then load them using OpenCV’s DNN module. This setup forms the backbone of our face detection system.
Analyzing and Marking Faces
We process the image to detect faces like this:
blob = cv2.dnn.blobFromImage(cv2.resize(image, (300, 300)), 1.0, (300, 300), (104.0, 177.0, 123.0))
net.setInput(blob)
detections = net.forward()
A “blob” is created from the image, which is a standard format for image processing in deep learning. We then pass this blob through the network to detect faces.
For each detection, we draw a bounding box around the face and label it with a confidence score:
for i in range(0, detections.shape[2]):
confidence = detections[0, 0, i, 2]
if confidence > 0.5: # if confidence is above 50%
box = detections[0, 0, i, 3:7] * np.array([w, h, w, h])
(startX, startY, endX, endY) = box.astype("int")
cv2.rectangle(image, (startX, startY), (endX, endY), (0, 0, 255), 2)
cv2.putText(image, f"{confidence * 100:.2f}%", (startX, startY - 10), cv2.FONT_HERSHEY_SIMPLEX, 0.45, (0, 0, 255), 2)
cv2_imshow(image)
This snippet cycles through all detections, filters them based on a confidence threshold, and then draws rectangles and labels on the image.
Building the Project
- Environment Setup: Install Python, OpenCV, imutils, and numpy.
- Capture Image: Use the
take_photo
function in a Colab notebook to capture an image with the webcam. - Process Image: Resize the image for optimal processing.
- Face Detection: Download the pre-trained model and use it to detect faces in the image.
- Display Results: Draw bounding boxes around detected faces and label them with confidence scores.
Learn How To Build AI Projects
Learn How To Build AI Projects
Now, if you are interested in upskilling in 2024 with AI development, check out this 6 AI advanced projects with Golang where you will learn about building with AI and getting the best knowledge there is currently. Here’s the link.
Conclusion
Face recognition technology is not just fascinating but also immensely useful in various sectors, from security to marketing. With Python, implementing face recognition is straightforward, providing a perfect blend of simplicity and power. This guide has walked you through each step to build a basic face recognition system. Now, it’s your turn to take this foundation and build upon it, exploring new possibilities and innovations in the world of computer vision and AI.