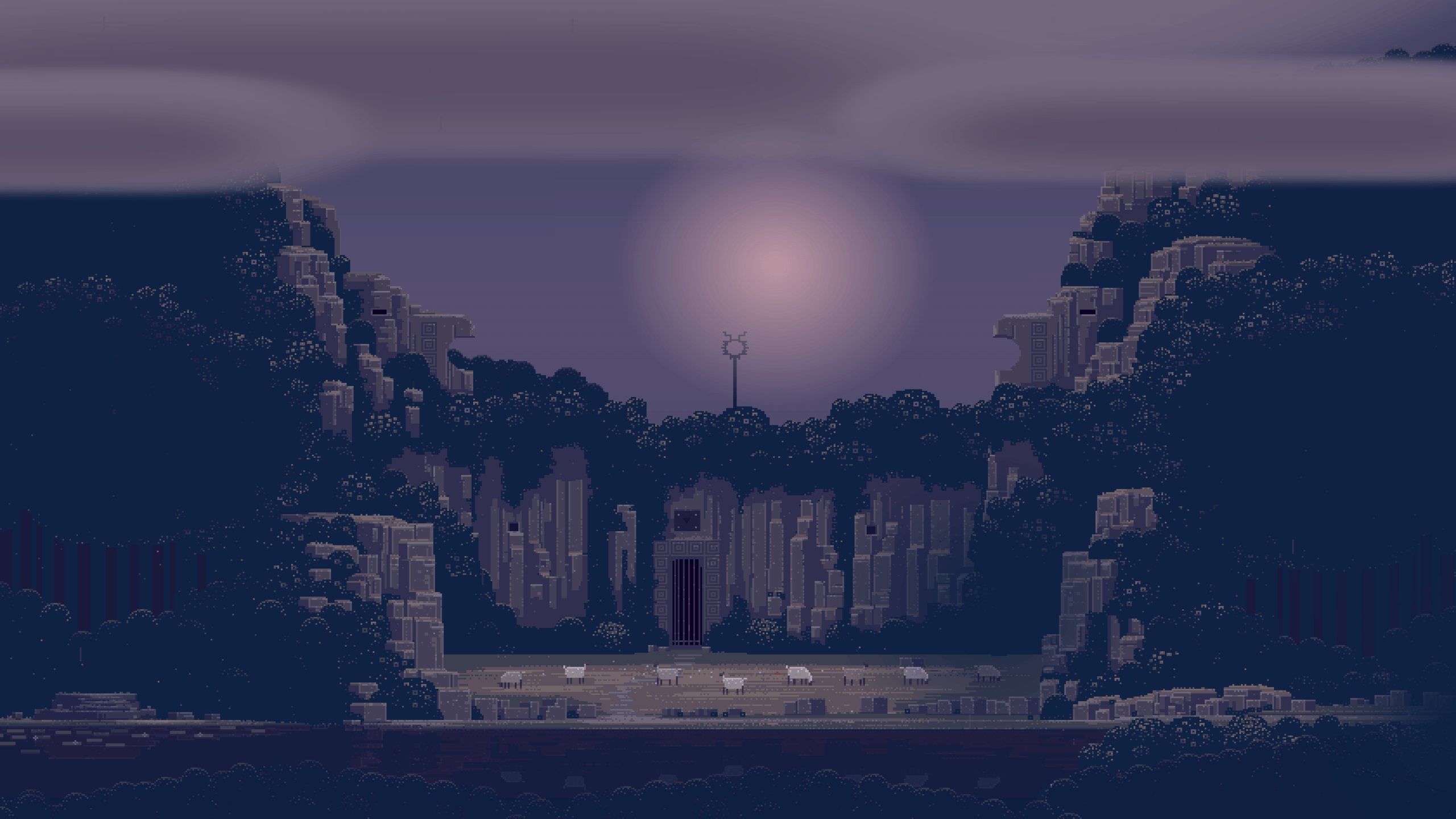
The RAG LangChain Tutorial represents a pinnacle in leveraging AI to transform unstructured data into actionable insights through a question-answering (QA) chain. This sophisticated process involves several key steps, from data ingestion to information retrieval and response generation, each underpinned by advanced coding and machine learning techniques.
Foundation: Setting Up the Environment Before delving into the functional aspects, establishing a secure and functional environment is crucial:
import os
api_key = os.environ.get('OPENAI_API_KEY')
In this snippet, the environment variable OPENAI_API_KEY
is retrieved, which is essential for authenticating and enabling the interaction with OpenAI’s API, laying the groundwork for the forthcoming operations.
Step 1: Loading Data - The Entry Point of Knowledge The first step in the QA chain is loading the data, which is pivotal as it forms the base of the knowledge system:
from langchain.document_loaders import WebBaseLoader
loader = WebBaseLoader("https://my.clevelandclinic.org/health/diseases/10946-cavities")
data = loader.load()
print(data)
Here, WebBaseLoader
is employed to fetch and load the document from a specified URL, converting unstructured web content into a structured LangChain Document. This transformation is crucial for enabling the systematic processing of data in the subsequent stages.
Step 2: Refining Data - Segmenting for Manageability Post loading, the document is segmented into manageable chunks, a process that enhances the system’s processing efficiency:
import tiktoken
tiktoken.encoding_for_model('gpt-3.5-turbo')
tokenizer = tiktoken.get_encoding('cl100k_base')
def tiktoken_len(text):
tokens = tokenizer.encode(text, disallowed_special=())
return len(tokens)
from langchain.text_splitter import RecursiveCharacterTextSplitter
text_splitter = RecursiveCharacterTextSplitter(chunk_size=100, chunk_overlap=20, length_function=tiktoken_len)
chunks = text_splitter.split_documents(data)
The tiktoken
library is crucial here, providing an encoding scheme compatible with the model in use, ensuring the text is appropriately segmented into tokens. The RecursiveCharacterTextSplitter
then processes these tokens, creating smaller text chunks or ‘splits’, optimizing them for the upcoming retrieval and processing phases.
Step 3: Vector Embedding Storage - The Core of Retrieval Storing the document splits in a vectorized form facilitates efficient search and retrieval:
from langchain.embeddings import HuggingFaceEmbeddings
model_name = "sentence-transformers/all-MiniLM-L6-v2"
hf = HuggingFaceEmbeddings(model_name=model_name, model_kwargs={'device': 'cpu'}, encode_kwargs={'normalize_embeddings': False})
embed = hf.embed_documents(texts=['h','e'])
from langchain.vectorstores import Chroma
vectordb = Chroma.from_documents(chunks, hf)
vectordb.similarity_search('bleeding gums', k=3)
HuggingFaceEmbeddings
generates vector embeddings of the document chunks, which are then stored in Chroma
, a vector database. This setup ensures that the system can quickly retrieve information relevant to user queries based on similarity metrics, showcasing a blend of NLP and vector search technologies.
Step 4: Intelligent Retrieval and Response Generation Combining the retrieval of relevant data with the generation of coherent answers is the final and most crucial step:
from langchain.chains import RetrievalQA
from langchain.chat_models import ChatOpenAI
llm = ChatOpenAI(model_name='gpt-3.5-turbo', temperature=0.6)
qa_chain = RetrievalQA.from_chain_type(llm, retriever=vectordb.as_retriever())
qa_chain({'query': 'How can I prevent cavity in my tooth?'})
The RetrievalQA
chain is a sophisticated construct that integrates the language model (in this case, ChatOpenAI
) with the retrieval system. It not only fetches pertinent information based on the query but also crafts a detailed and contextually accurate answer, embodying the essence of an AI-driven QA system.
Extending the Conversation: Enabling Multi-Turn Dialogues The tutorial also hints at the possibility of extending this QA system to support multi-turn conversations, adding a layer of complexity and user engagement. This involves maintaining a contextual memory of the conversation, allowing the system to provide more relevant and nuanced responses over time.
Comprehensive Overview: Unpacking the Mechanisms of AI-Driven QA Through each step of the RAG LangChain Tutorial, from data loading and splitting to embedding, retrieval, and generation, we unravel the intricacies of building a robust QA system powered by AI. The code not only serves as a functional guide but also as a conceptual framework, demonstrating how different components of AI and machine learning can be harmoniously integrated to create intelligent, responsive systems capable of processing vast amounts of unstructured data to generate meaningful answers.
In summary, the RAG LangChain Tutorial offers an exhaustive exploration into the construction of an AI-driven QA system, highlighting the sophisticated integration of technologies like LangChain, HuggingFace, and OpenAI. Each code segment is meticulously crafted to contribute to the overarching goal of transforming raw data into a rich, interactive dialogue platform, paving the way for future advancements in AI and machine learning applications.
Learn How To Build AI Projects
Learn How To Build AI Projects
Now, if you are interested in upskilling in 2024 with AI development, check out this 6 AI advanced projects with Golang where you will learn about building with AI and getting the best knowledge there is currently. Here’s the link.